[TOC] #### 1. JS 正则表达式 --- JS 正则表达式语法: ``` # JS 的正则表达式不需要使用引号包裹,PHP 需要使用引号包裹。修饰符是可选的,可写可不写 /正则表达式主体/修饰符 ``` JS 中使用正则表达式的方法比较多,可以按照使用两种类型记忆: 字符串对象方法、正则表达式对象方法 ```javascript // 字符串对象方法 string.search(regexp) // 正则表达式对象方法 regexp.test(string) ``` #### 2. 使用字符串方法 --- `string.search(regexp)` 匹配首次出现的下标 ```javascript const string = 'hello world !' // 返回内容首次出现的位置(下标),没有匹配到时返回 -1 const index = string.search(/world/) ``` `string.replace(regexp, new_string)` 将首次匹配到的内容进行替换 ```javascript const string = 'hello world !' // 将首次匹配到的内容进行替换 const result = string.replace(/world/, 'vue') ``` `string.match(regexp)` 执行正则表达式匹配 ```javascript const string = 'hello world !' // 下面 result1 和 result2 结果相同 // ['world', index: 6, input: 'hello world !', groups: undefined] const result1 = string.match(/world/) const result2 = /world/.exec(string) ``` `string.matchAll(regexp)` 执行正则表达式匹配,匹配字符串所有符合条件的内容 ```javascript const string = 'hello world world !' const result = [...string.matchAll(/world/g)] console.log(result); ``` 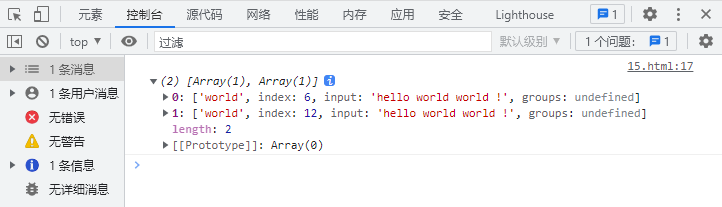 #### 3. 使用 RegExp 方法 --- `regexp.test(string)` 用于检测一个字符串是否匹配某个模式 ```javascript const string = 'hello world !' const bool = /world/.test(string) ``` `regexp.exec(string)` 执行正则表达式匹配,匹配成功时返回一个数组,匹配失败返回 null ```javascript const string = 'hello world !' // ['world', index: 6, input: 'hello world !', groups: undefined] const result = /world/.exec(string) ```